On Adobe’s Tools and Services page there is an intriguing remark about the company’s plans for a code editor. “We think there’s a need for a different type of code editor – we’re working on something and will have more to share soon.”
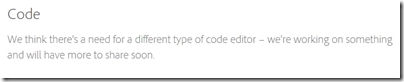
That something is Brackets, a code editor written in HTML and JavaScript (which means, as with all the best tools, that you can code Brackets in itself).
Advertisement
Although Brackets is written in HTML and JavaScript, it is not yet a web application. Instead, it runs on the desktop using Google’s Chromium Embedded Framework (CEF), which lets you embed the Chrome (strictly, the Chromium) browser engine in a desktop application. In the case of Brackets, the wrapper is lightweight, the intention being that in future Brackets may be fully browser-hosted. The consequence though is that currently you need Google Chrome installed and it only runs on Windows and Mac.
The project is open source under the MIT license; anyone can grab the code from Github. Brackets also depends on another open source project, CodeMirror, which is a JavaScript editor component for browsers. I installed it on Windows and soon had it up and running. Note that you should pull brackets_app if you want to run it, as this brings down the Brackets code as well.
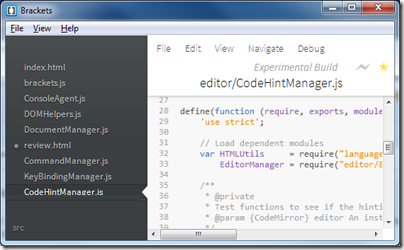
I spoke to Brackets Product Manager Adam Lehman. “This might be the first project we started with the intent of being open source from day one,” he said.
“Our general intent is that we wanted to provide an editor that web developers felt that they could own. In the past we might have built something in Eclipse, and there would have been this giant gap between the person who knew HTML, JavaScript and CSS, and then having to write a Java-based Eclipse plug-in to extend the editor.
“When we start talking to developers, they’re going back to just text editors, things that don’t do much more than edit and manage a document, and as complex as HTML and JavaScript apps are getting these days, it seemed crazy that our tools weren’t keeping up with us. So the idea was to start this project, add a little bit of our own ideas, and have the community supply their own ideas.”
But how does Adobe intend to use Brackets in its own products, and what is the business model?
“We believe there are two spaces for the editor market. There is the larger IDE, but there’s also these lightweight text editors. We’re finding that the traditional JavaScript and HTML developer, CSS developer, was heading towards the lightweight text editor and not towards the larger IDE. We don’t see Dreamweaver and Brackets as direct competitors because they service two different tastes. It wasn’t a matter of could we add a feature here or there that was going to get people to use Dreamweaver. It was that difference between our larger tool and a much lighter weight tool. That’s where Brackets come in.”
How then will Brackets tie in with other Adobe products?
“That’s the key for Brackets. We wanted to see if we could innovate in the space and we also wanted to have a common language that we could start targeting. When you say, I want to open up an editor from Adobe Edge and start coding, we needed to define what that editor would be, so Brackets would come into that.
“We’re also saying we need to do better tooling around PhoneGap. A lot of people are fine with the command line, but we want to take a step beyond that and so Brackets is the obvious place where we’ll start to build an extension where we can tie into PhoneGap Build, or extensions around the PhoneGap APIs.
“We’ve got a lot of ideas around using Brackets to bring a lot of our HTML efforts together, not only our core HTML products but also a lot of the W3C and WebKit work that we’re doing. Brackets is a great place to put tooling, that isn’t quite ready for mass consumption yet, but we could actually build extensions for something like Shaders where those people who are interested in it can get in and start playing around with it.
“The beauty of building on the web platform is that we can go wherever the web platform goes.”
Initial prototypes of Brackets ran entirely in the browser, which would be interesting for future versions of Adobe’s Creative Cloud as well as other scenarios, but Lehman said this got mixed reactions.
“While we believe that the future of development is heading towards the cloud, and the general consensus from developers is the same, we also heard that it is not ready yet. We decided to focus on a desktop version first, with the idea that towards the end of this year or beginning of next year we’ll start to supplement with other targets, whether it be in the cloud or a tablet, or embedded in a tool like Edge,”
says Lehman. He adds that a browser-hosted Brackets could end up integrated with the PhoneGap Build site. PhoneGap Build is a service for compiling cross-platform HTML and JavaScript into mobile apps for a variety of devices.
Since Brackets is built on Google’s Chrome/Chromium platform, what are the implications for cross-browser compatibility?
“There’s two pieces to it. There’s our container that Brackets runs in, and that is running on Chromium.
“The other part is that we have this live preview system which is tied directly to Chrome on the desktop. We happened to just start with Chrome, mostly because there is a remote debugging API that’s pretty fleshed out there.
“With Firefox and Internet Explorer, it’s a little bit different. We talked to Mozilla and they’re just now starting to work on that remote debugging API and trying to get it inline with where Chrome is, so we’re expecting to hear from them in August that they actually might have an API where we can start to build that same functionality, which is our intent.
“We’ve already started engaging with Microsoft about Internet Explorer. Right now their remote debugging API is somewhat private and in the form of a COM object which is not ideal coming from a JavaScript perspective, but we’ve showed them what we’re after, and we’ve started discussion of what a remote API from IE might look like that didn’t require COM. We’re exploring those options. Those are our priorities right now.
“If we build a cloud-based version then it’s going to be a question of what browsers this is going to run in. Our intent is to run in the modern major browsers. We aren’t building anything that’s Chrome-specific, we’re doing our best to stay as browser-agnostic as we can, but we are likely to require a more modern browser. We feel it would be OK to require the latest versions of Firefox, IE or Chrome.”
I asked Lehman whether Brackets might be useful for server-side as as client-side code. He said that Brackets is focused on the client, though a community extension is under way for node.js. He adds that since Brackets is fully extendable, others may do plug-ins for languages such as PHP.
Why is Brackets at Github and not Apache?
“We have a lot of people at Adobe who work for Apache now, and we talked to them before we released Brackets. Our sense is that Apache might be too much of a turn-off for the individual contributor, who just wants to hack and fix a bug and submit it back,”
Lehman told me. Although there are external contributors, all the committers are currently at Adobe, though there are plans for adding external committers by the end of the year. “We don’t want this to be 100% Adobe controlled.”
When will Brackets get to version 1.0?
“We’re being as agile as we can. Every bit we add, it comes closer to being a 1.0 for somebody. The things that I think are missing that you would expect out of a core editor are around code-completion for CSS and JavaScript, and solid and advanced search and replace. In the web world, that’s how we refactor code. We’re hoping to drive those in by November time. But we are on 2.5 week sprints and things change rapidly.”
I also asked about plans for a mobile app version of Brackets. Lehman says that is planned for next year, though the community is working on getting a Linux version working and support for ChromeOS.
Brackets is a fascinating project on several levels. What stands out is how far Adobe has moved from being the Flash company. A few years back Adobe came up with a system for having Flash applications run on the desktop and on mobile devices: Adobe AIR. It also invested in Eclipse and came up with the Flash Builder IDE.
Now here is Adobe with an open source project for a desktop application built from HTML, JavaScript, and a third-party open source browser engine; and in place of mobile AIR it has PhoneGap.
It is a big change, most of which has become publicly known in less than a year, signalled by the repositioning of Flash and AIR versus HTML in September 2011, and the abandonment of Flash for Mobile in November 2011.
As for Brackets itself, it is well worth a look though probably not a tool you want to use for real work just yet. In a few months though, that may well change.
Postscript: Brackets reminds me of another Adobe, or rather Macromedia, HTML code editor. That was HomeSite, an excellent text-based tool that Adobe discontinued in 2009; active development ceased years before that.